Lately I’ve been hearing a lot about ChatGPT, an AI that takes and answers prompts from a user. Perhaps the best way to describe ChatGPT is to just ask it, and here’s the answer given to me by the AI: “ChatGPT is a great tool for developers who want to create powerful AI-powered chatbots. It is easy to use and requires minimal coding knowledge. With ChatGPT, developers can create chatbots that can understand and respond to natural language queries with natural language responses. This makes it possible to create chatbots that can provide an engaging and interactive experience for users.”
After watching a few videos I was fascinated, and looked around quickly for a tutorial on how to set it up on my computer. After a quick search I found an article that described how to set it up, and I was impressed with how straightforward it is! If you decide to skip the tutorial linked and just copy the code, remember you need to first ‘pip install openai’ in your terminal to install the package.
So, after slamming it with prompts all morning to test its capabilities, I’m impressed. It’s not perfect – for example it gave me wrong answers when I asked it to find the source for some random quotes I pulled from a book, but on the whole it did pretty well for itself. Now I want to show you two little programs I threw together. Even as a completely novice programmer, this AI lets you do some pretty sophisticated tasks.
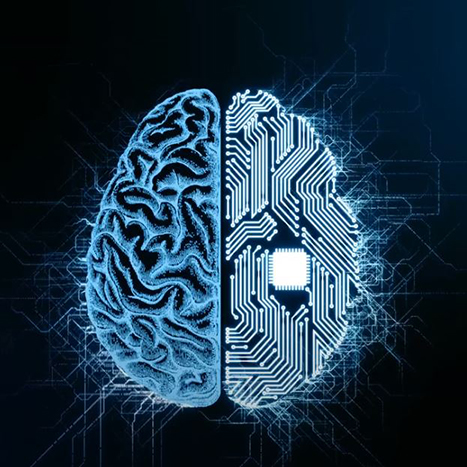
A ‘This Day in History’ generator:
Working from the base code offered in the tutorial in order to set up ChatGPT, what I was next interested in was essentially creating a little extra code that interacted with the ‘Prompt’ variable. First I thought it would be fun to ask it “List what happened in history on [today’s date]”, sans the year, which would just have it tell us what happened this year. So let’s start with getting that date:
import openai
from datetime import datetime
today = datetime.today()
# convert to date String
date = today.strftime("%m/%d")
Now, you just need to be able to plug that date into the prompt being fed to the AI:
prompt = "List what happened in history on "+str(date)
When given the prompt, the AI returns this:
- In 1788, the first British penal colony was established in Australia.
- In 1848, the Treaty of Guadalupe Hidalgo was signed, ending the Mexican-American War.
- In 1887, the Dawes Act was passed in the United States, which divided Native American tribal lands into individual allotments for Native Americans.
- In 1933, the 20th Amendment to the United States Constitution was ratified, changing the date of the beginning and end of presidential and congressional terms.
- In 1945, the Soviet Union and Poland signed the Yalta Agreement, which established the post-World War II borders in Eastern Europe.
- In 1974, the Symbionese Liberation Army kidnapped 19-year-old Patricia Hearst in Berkeley, California.
Pretty neat! Here’s the whole block of code:
import openai
from datetime import datetime
today = datetime.today()
# convert to date String
date = today.strftime("%m/%d")
openai.api_key = "YOUR KEY GOES HERE"
model_engine = "text-davinci-003"
prompt = "List what happened in history on "+str(date)
completion = openai.Completion.create(
engine=model_engine,
prompt=prompt,
max_tokens=1024,
n=1,
stop=None,
temperature=0.5,
)
response = completion.choices[0].text
print(response)
A Quote Analyzer
The bot’s performance analyzing quotes was… Really pretty amazing. My idea was that I would basically feed it the prompt “Analyze this quote: [quote]”, give it some philosophy, and see what it told me. The quote I gave it was
“Remember that you are an actor in a play, which is as the playwright wants it to be: short if he wants it short, long if he wants it long. If he wants you to play a beggar, play even this part skillfully, or a cripple, or a public official, or a private citizen. What is yours is to play the assigned part well. But to choose it belongs to someone else.”
The analysis provided:
“This quote is suggesting that we should accept our roles in life and make the best of them. We may not be able to choose our circumstances, but we can choose how we react and respond to them. The quote encourages us to be resilient, take responsibility for our actions, and strive to do our best in all aspects of life.”
To make this a little easier to do from the base code, I just had Python ask for an input, where I would place the quote, and then fed that to the prompt generator, like so:
promptin = input('What quote would you like to analyze?\\n')
prompt = "Analyze this quote: "+str(promptin)
The whole code looks like this:
import openai
openai.api_key = "YOUR KEY GOES HERE"
model_engine = "text-davinci-003"
promptin = input('What quote would you like to analyze?\\n')
prompt = "Analyze this quote: "+str(promptin)
completion = openai.Completion.create(
engine=model_engine,
prompt=prompt,
max_tokens=1024,
n=1,
stop=None,
temperature=0.5,
)
response = completion.choices[0].text
print(response)
This is only two ideas of what you could do with this generator – the beauty of it is that the AI itself is doing pretty much all the heavy lifting, and all we’re doing is tampering with the prompt in various ways. You could get this to give you a quote of the day every day, or even help you plan out your tasks, if you have a good way to feed the prompt with that data.