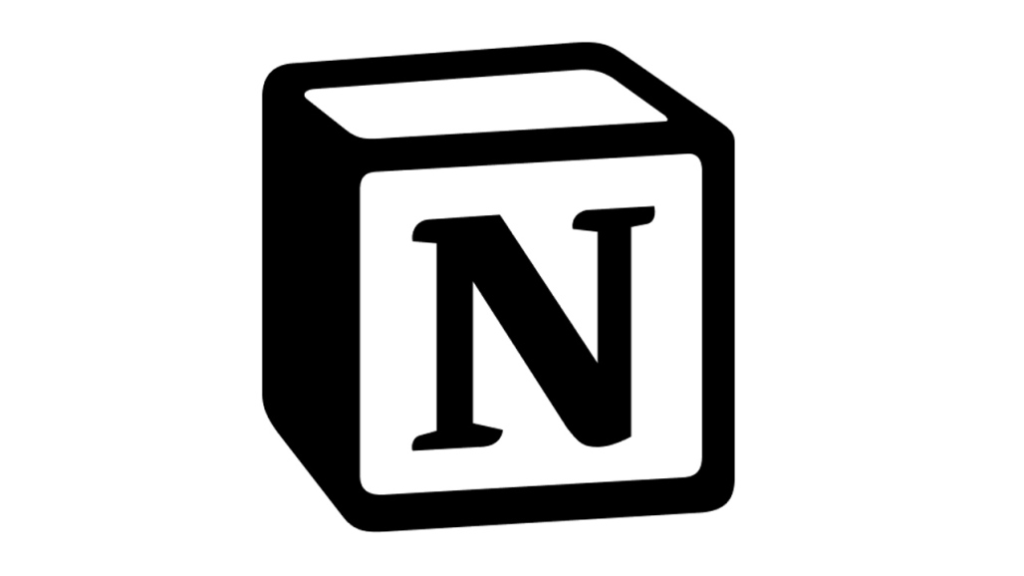
Today I want to talk about a little trick to add some extra functionality to Notion. Many of you might have already played with interesting web-hosted embeds – by typing “/embed”, you can embed web-pages into a Notion page, and this has lead to some pretty creative tools already. Things like embedding weather widgets into dashboards, spotify playlists, the like. Apption.co actually has its own “DIY Embed URL” tool, as well, where you can host your own HTML instead of using a local host, which I’ll be using in this example.
My case study is going to be how you can make your own simple little HTML tool to add some extra functionality to Notion. This one will randomly selects an item from a JSON list – we’re then going to embed it into Notion.
Lately, I’ve been playing with a new idea. Some of you might have read a previous article I wrote on creating a “Rewards” system in Notion. In my usual fashion, I’m constantly messing with these systems to see if I can make them more interesting or effective. Neuroscientist Andrew Huberman has made the interesting observation in a few of his podcasts on a phenomenon known as “Reward Prediction Error”, which are essentially differences in the amount of dopamine – a neurotransmitter responsible for motivation – that we receive from rewards when they are unpredictable. It turns out, curiously enough, that the inconsistent rewards can actually be more motivating. This is mechanically what’s behind the vast appeal of gambling at casinos, and RPG loot obsession. So I thought: what if I made a little intermittent reward slot-machine, a button I could click which would randomly select a reward – or often, not – and my work essentially earned me clicks on this dystopian self-imposed Skinner box? Sounds like a fun experiment. You might be more interested in it generating a random quote, or simply just a random number, like a dice roller – all would work on the same principle.
The Code:
If you’re not savvy with HTML, don’t fret. We live in a magical world of AI superintelligence, where you can just ask a robot to slap it together for you in a couple of seconds… Which is what I did. Shame on me, I know. Prompting ChatGPT for such a tool produced me these results, and with a little tinkering, the HTML was in good shape for embedding into Notion. I use dark mode, so I set a transparent background and white font – keep that in mind. On a white background, you’re gonna have trouble seeing.
So let’s start by creating a .html file in a new empty folder, and pasting in this code.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Random Item Selector</title>
<style>
button {
font-size: 16px;
padding: 10px 20px;
background-color: gold;
color: black;
border: none;
border-radius: 4px;
cursor: pointer;
}
button:hover {
background-color: #ffa500;
}
#result {
font-size: 24px;
font-weight: bold;
margin-top: 20px;
color: white;
}
body {
background-color: transparent;
}
</style>
</head>
<body>
<h1 style="color: white;">Random Item Selector</h1>
<button onclick="selectRandomItem()">Select Random Item</button>
<div id="result"></div>
<script>
function loadItems() {
const items = [
'Item 1',
'Item 2',
'Item 3',
'Item 4',
'Item 5'
];
return items;
}
async function selectRandomItem() {
const items = loadItems();
const randomIndex = Math.floor(Math.random() * items.length);
document.getElementById('result').innerText = items[randomIndex];
}
</script>
</body>
</html>
Replace ‘Item 1’ through ‘5’ with whatever you want, or add more in the same format: it’s gonna pick one at random. In my configuration of rewards, in keeping with the spirit of “Reward Prediction Error” and a bit of masochism, I also included a bunch of “Nothing” items. If you were a little more sophisticated, you could build percent chances right into the code rather than having like seventy “Nothing” values in your array like a barbarian, but hey, if it works it works!
Hosting locally:
The last step will be hosting it. To host it locally you’ll want Python installed – if you don’t, and don’t want to bother, scroll down to the “Hosting using Apption.co” section below. If you want to host it locally, navigate to your directory, and run this command in the terminal:
python -m http.server
This is going to host your directory at this URL: http://localhost:8000/
Now if you click on your HTML in this new directory, you’ll see your generator. Since I modelled it for dark mode, it looks like this
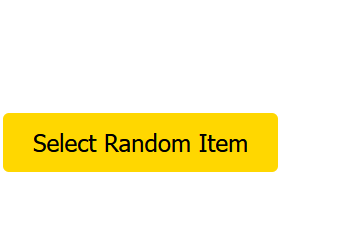
But if you take that localhost URL and paste it in as an /embed link, then click the button, it’ll look like this:
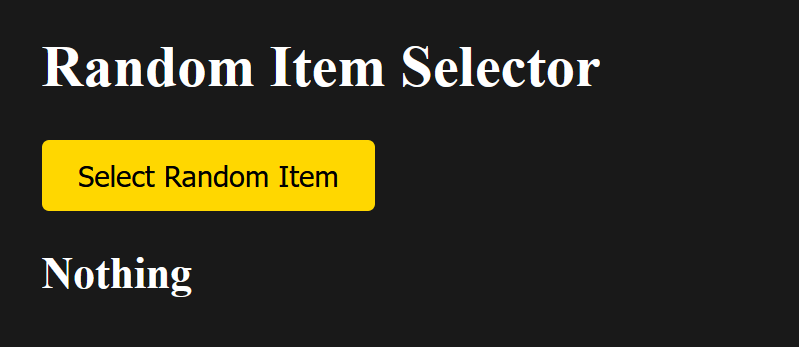
… Bad luck on my Skinner box. Keep in mind, though, that if you stop hosting your server, it’ll stop working. This is annoying. If you own a domain, you can host your embed on your own domain, but if you don’t, there’s an alternative.
Hosting using Apption.co
If you want to take local host out of the equation, you can create an account at Apption.co and go to “DIY Embed”, you can paste HTML when prompted. Apption has a limit of embeds on their free plan, however, so keep that in mind.
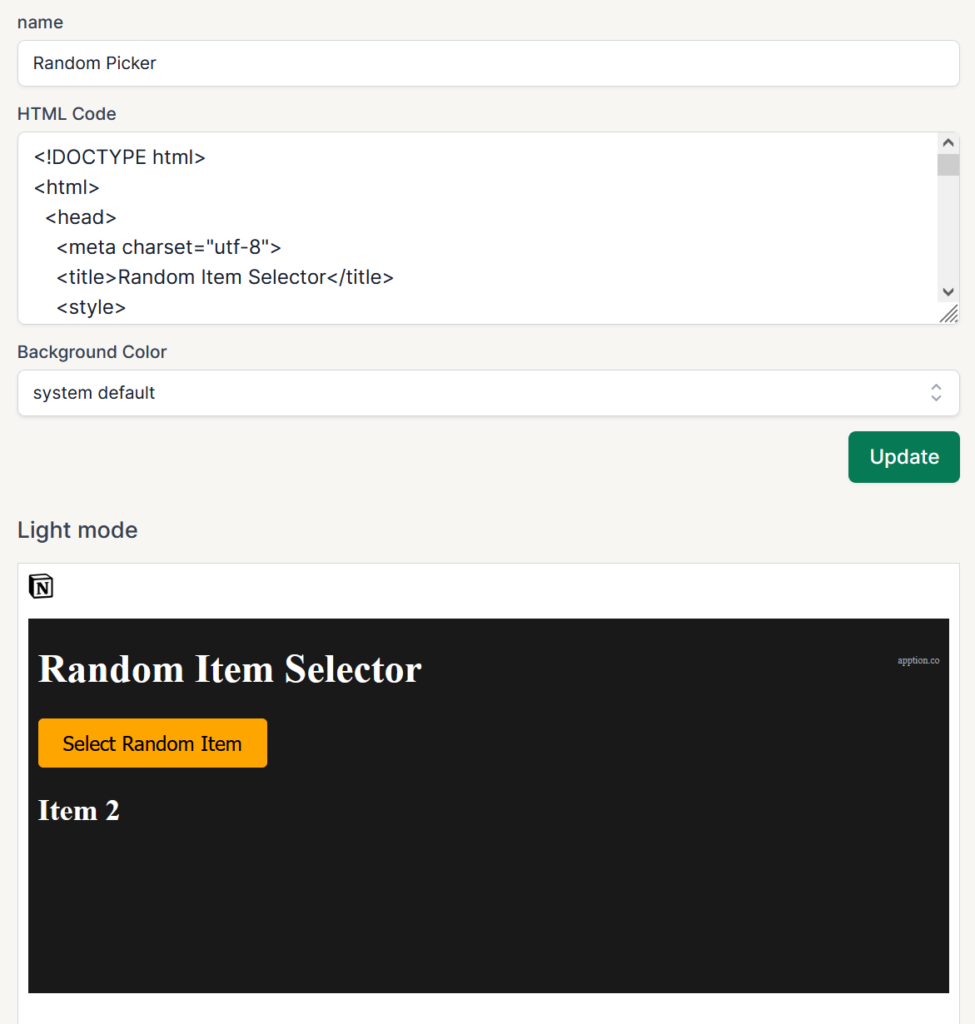
They’ll provide you with an embed URL, which you’ll paste into Notion’s “Embed”, and there you go!
There are a lot of possibilities with Notion embeds, and AI tools like ChatGPT are making them increasingly accessible to coding amateurs. I’d love to see if any of you guys come up with interesting ideas for other embeds!